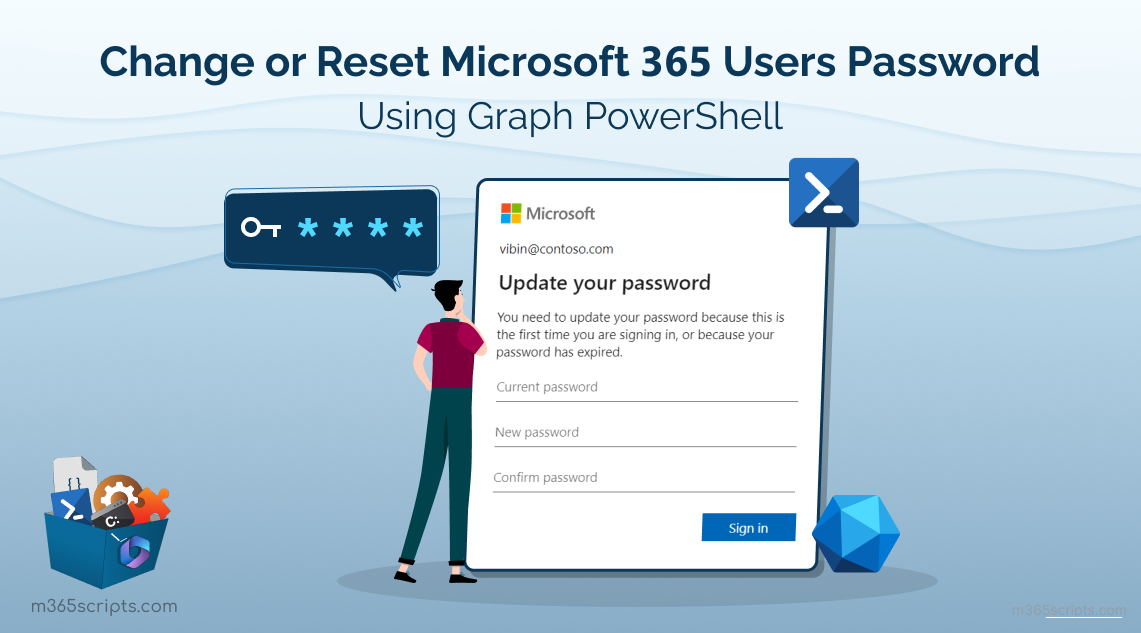
Manage Microsoft 365 User Passwords Using Graph PowerShell
One critical aspect of managing Microsoft 365 users is ensuring the security of their accounts, and a key component of this is efficient password management. To prevent unauthorized access and maintain account security, it is essential to manage Microsoft 365 user passwords.
In user password management, PowerShell stands out over Microsoft 365 admin centers by automating tasks and enabling efficient bulk operations. It also offers high customization to align with specific organizational password policies and compliance requirements.
In this blog, we’ll delve into discussing the PowerShell operations that help admins effortlessly manage Microsoft 365 user passwords.
The following list outlines major PowerShell operations to manage Microsoft 365 user passwords:
- Reset user passwords in Microsoft 365
- Reset user passwords with a temporary one
- Force M365 users to change their password
- Bulk reset passwords in Office 365
- Disable strong passwords for M365 users
- Set M365 user’s password to never expire
- Check the last password change date and time
- Check password expiration date for users
Before proceeding, ensure to connect to the Graph PowerShell module using the global admin account with the following scopes:
- User.ReadWrite.All
- Directory.AccessAsUser.All
- UserAuthenticationMethod.ReadWrite.All
Note: To manage user passwords with PowerShell, we prefer the MS Graph module because cmdlets from Azure AD and MS Online module are being deprecated.
Follow these guidelines when creating passwords or configuring password policies in your Microsoft 365 environment.
- New passwords must be between 8 and 256 characters and use a combination of at least three of the following: uppercase letters, lowercase letters, numbers, and symbols.
- This requirement ensures the creation of a robust and strong password. Failure to adhere to these criteria may result in execution errors.
- Also, always ensure to follow essential security best practices for your Microsoft 365 and update password policies to stay ahead of cybersecurity threats.
In the Microsoft Graph PowerShell module, you can use the “Update-MgUser” cmdlet to update user passwords. This operation is especially helpful when a user approaches the admin to reset a forgotten Microsoft account password. Here’s a demonstration to change the user password:
$password = @{ Password = “<Password>” ForceChangePasswordNextSignIn= $false } Update-MgUser -UserId “<UserId Or UPN>” -PasswordProfile $password
Executing the above snippet with the appropriate user id/UPN and password will change the user password in Microsoft 365.
You can assign a new temporary password for a Microsoft account and enforce a password reset on the next login. Here’s a snippet to do such a thing.
$password = @{ Password = “<Password>” } Update-MgUser -UserId “<UserId Or UPN>” -PasswordProfile $password
After execution, when the designated user attempts to log in with the provided password, they will be prompted to reset it through the graphical user interface (GUI). This ensures a secure and user-friendly approach to password management in Microsoft 365.
To force a Microsoft 365 user to reset their password on the next login, without using a temporary password, employ the following approach with the “Update-MgUser” cmdlet:
$password = @{ ForceChangePasswordNextSignIn= $true } Update-MgUser -UserId “<UserId Or UPN>” -PasswordProfile $password
This case becomes necessary after creating bulk users using the Microsoft 365 admin center, as the temporary password gets generated at the time of creation itself.
To reset passwords for multiple users in Microsoft 365 using PowerShell, create a CSV file with the user’s UPN and the new password.
After the creation of the CSV file, just execute the script below based on your requirements. Change the password profile hash table to meet your needs.
Password profile hash table | Description |
Password = “<Password>” ForceChangePasswordNextSignIn= $false |
Resets user password and neglects any prompt to force the user to reset on the next sign-in. |
Password = “<Password>” |
Resets the user password and forces them to update the password on the next sign-in. |
ForceChangePasswordNextSignIn= $true |
Just forces the users to update the password on the next sign-in without a temporary password allocation. |
Import-CSV “<File Location>” | ForEach-Object { $password = @{ #Replace this hash table keys and values as per your requirements ForceChangePasswordNextSignIn = $false Password = $_.NewPassword } $currentUPN = $_.UPN try { Update-MgUser -UserId $currentUPN -PasswordProfile $password -ErrorAction Stop Write-Host -ForegroundColor Green "Password updated for $($currentUPN)" } catch { $errorMessage = $_.Exception.Message Write-Host -ForegroundColor Red "Failed to update the password for $($currentUPN): $($_.Exception.Message)" } }
Replace the <FileLocation> with the appropriate saved location of the CSV file.
Note: If you want to reset the password for all users with the same password, omit the ‘NewPassword’ column in the CSV file and directly assign the password string in the hash table.
With the Microsoft Graph PowerShell module, you can effortlessly disable the strong password requirement for a user by updating the ‘PasswordPolicies’ property. Executing the command below will adjust the password policy and disable password complexity in Microsoft 365 for the specified user.
Update-MgUser -UserId “<UserId Or UPN>” -PasswordPolicies DisableStrongPassword
To revert this setting and re-enable the strong password requirement for the user, use the following command:
Update-MgUser -UserId “<UserId Or UPN>” -PasswordPolicies None
For bulk user operations, create a CSV file with the header “UPN” and execute the cmdlet as demonstrated below:
Import-CSV “<FileLocation>” | ForEach-Object {Update-MgUser -UserId $_.UPN -PasswordPolicies DisableStrongPassword}
Sample Input File:
Warning: Disabling the strong password requirement in Microsoft 365 may compromise security. Therefore, careful consideration and the implementation of alternative security measures, such as multi-factor authentication configuration, are strongly advised.
You can set the user’s password to never expire if the password expiration policy is configured in your Microsoft 365 tenant. To set an individual user’s password to never expire using Graph PowerShell, utilize the below cmdlet.
Update-MgUser -UserId “<UserId Or UPN>” -PasswordPolicies DisablePasswordExpiration
This ensures a seamless user experience without the need for periodic password changes.
The last password change date and time of an M365 user can be checked with the help of the following PowerShell cmdlet.
$LastPasswordChangeDateTimeDetails = Get-MgUser -UserId “<UserId Or UPN>” -Property DisPlayName, UserPrincipalName, PasswordPolicies, LastPasswordChangeDateTime $lastPasswordChangeDateTimeDetails | Select DisPlayName, UserPrincipalName, PasswordPolicies, LastPasswordChangeDateTime
To check the password expiration date for a user using Graph PowerShell, use the following cmdlet:
(Get-MgUser -UserId "<UserId Or UPN>" -Property UserPrincipalName, lastPasswordChangeDateTime).lastPasswordChangeDateTime.AddDays(90)
Note: This execution calculates the password expiration date based on the user’s last password change timestamp and concerning the default password expiration policy (90 days). If your tenant has a different expiration policy, update the number of days in the cmdlet instead of “90” to get the desired output.
To export all user’s password expiration and last password change dates, you can utilize this prebuilt PowerShell script that generates seven different types of reports. As this script calculates password expiry date based on the domain’s password expiration policy, it eliminates concerns about custom policies configured.
Also, in the case of self-service password scenarios, you can ask the users for “Face Check” verification to ensure the right user. It can be done also for password reset cases by help desk services. Furthermore, you can disable the ‘Stay signed in?’ prompt that appears after the authentication window, to prevent users from remaining signed in.
While the above approach proves efficient for password management, it’s crucial to acknowledge that effectively monitoring password activities can be challenging. Crafting PowerShell scripts and applying filters on cmdlets for M365 password reports may feel distressing, especially for beginners.
AdminDroid is here – the ultimate savior for streamlining administrative tasks. Offering an in-depth exploration of every password activity with a granular view, AdminDroid eliminates the need for intricate scripting. Here are some of the Microsoft 365 password reports provided by AdminDroid, each designed to leave you impressed:
- Microsoft 365 password-expired users
- Users with soon-to-expire password
- M365 users with password never expire
- Users who never changed their password
- Users have not changed passwords in 90 Days
- Recently password-changed users
- Users allowed to have a weak password
- Password policies applied in your tenant
From a drone-shot-like perspective, AdminDroid’s password dashboard gallery offers a comprehensive overview of password statistics, combining insights from the reports mentioned above.
Beyond password reports, AdminDroid’s Azure AD reports offer invaluable insights into user properties, external users, licenses, and MFA statuses. Overwhelming the native tools, this functionality empowers admins to monitor Azure AD information with nicely formatted reports.
The Azure AD auditing tool by AdminDroid allows admins to closely monitor changes in user profiles, logins, MFA, passwords, groups, and admin roles, providing real-time updates to fortify organizational security.
Here’s the surprise! You may find it hard to believe, but AdminDroid Azure AD management allows free access to 120+ reports for MS Entra auditing and reporting.
AdminDroid doesn’t stop at Entra ID management; it extends to and connects with all Microsoft 365 services for comprehensive reporting. In total, the tool offers an impressive collection of 1800+ reports and 30+ dashboards, all accessible with a 15-day premium edition.
Download the AdminDroid M365 reporting tool today and simplify Microsoft 365 management effortlessly!
Overall, we have aimed to provide comprehensive guidance to reset and manage Microsoft 365 user passwords using PowerShell. Contributing to user management in Microsoft 365, we trust this blog serves as a valuable resource to strengthen user password protection. Your thoughts and comments are highly encouraged; feel free to share your insights in the comments section.