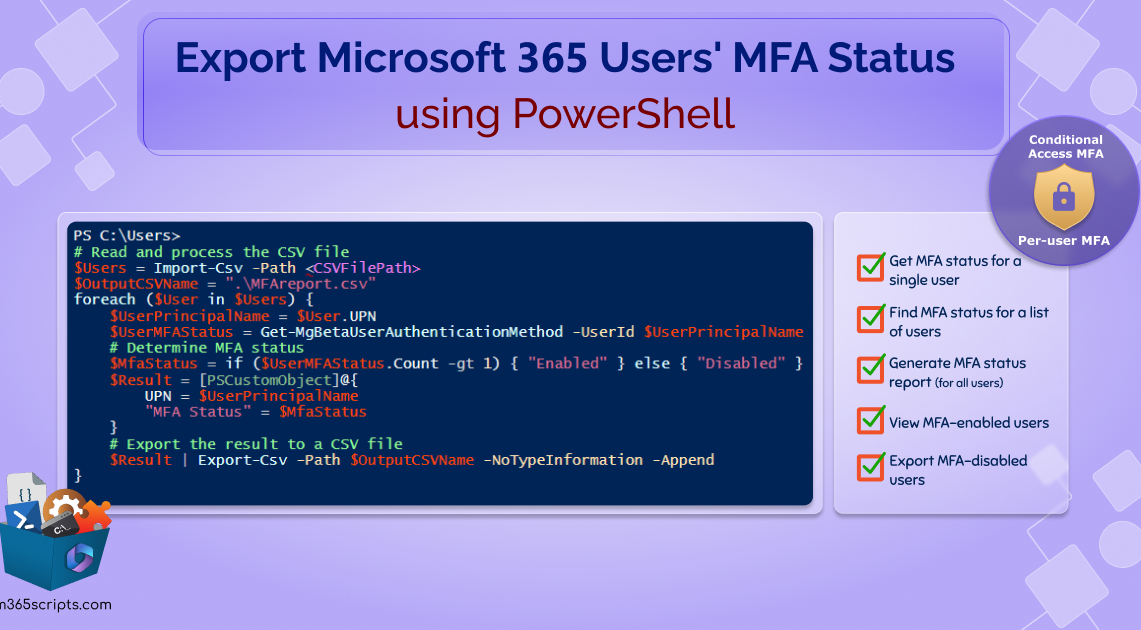
Check MFA Status for Microsoft 365 Users using PowerShell
Configuring Multi-Factor Authentication (MFA) is pivotal in safeguarding Microsoft accounts from compromises. MFA can be configured through various methods, such as per-user MFA, security defaults, and Conditional Access policies. Admins can select the appropriate method based on their organization’s requirements and licensing constraints. Also, monitoring MFA status of all users ensures that this security control is effectively implemented and maintained across the organization.
Retrieving the MFA status of Microsoft 365 users can be a bit of a puzzle. Two primary methods are commonly employed for this purpose: the MSOnline module and the MS Graph PowerShell module. However, both approaches come with their own set of challenges.
1. MSOnline Module (Get-MSolUser)
The MSOnline module, while still in use, is on the path to deprecation and will no longer be supported after March 2024. This module is effective mainly when your organization relies on legacy per-user MFA. It provides relatively straightforward MFA status information.
Note: Microsoft suggests converting from per-user MFA to CA policies for improved security.
2. MS Graph PowerShell Module (Get-MgUserAuthenticationMethod)
The MS Graph PowerShell module offers a more future-proof solution. However, it introduces complexity in determining MFA status. Unlike the MSOnline module, it does not directly display the MFA status as “enabled” or “enforced.” Instead, it requires administrators to consider the authentication methods to decide MFA status.
Additionally, a notable drawback of the MS Graph approach is that even if MFA is disabled, the authentication methods for a specific user can still appear active. This can lead to inaccuracies when attempting to ascertain MFA status based solely on authentication methods. I hope Microsoft will provide a solution for this issue soon.
So, I have given both ways to check MFA status using Get-MSolUser and Get-MgUser. You can choose based on your needs.
Before running the PowerShell scripts, you must connect to Microsoft Graph PowerShell or MsOnline PowerShell module. This blog covers various use cases related to exporting MFA status reports:
- Get MFA status for a single user
- Get MFA status for a list of users
- Generate MFA status report (for all users)
- View MFA-enabled users
- Export MFA-disabled users
Note: The exported file will be stored in the current working directory.
Often, admins want to check if MFA is enabled for a specific user, and here is a simple code to find that.
MS Graph:
To view the MFA status for a single user using Microsoft Graph, run the below cmdlet.
Get-MgBetaUserAuthenticationMethod -UserId <UPN> | select *
Every user account will have a password authentication method by default. If the cmdlet lists more than one authentication method, you can conclude that MFA is enabled for that user.
Here, the user has 2 MFA authentication methods. So, we can say that the user is protected by MFA.
MSOnline:
If you’d like to use MSOnline module, execute the below cmdlet.
(Get-MsolUser -UserPrincipalName <UPN>).StrongAuthenticationRequirements.State
If the cmdlet returns ‘Enabled’ or ‘Enforced,’ MFA is enabled for that user. If it doesn’t return any result, MFA is disabled for that user.
Administrators can efficiently retrieve MFA status report for a list of users by importing a CSV file.
MS Graph:
To view MFA status for bulk users using MS Graph PowerShell, run the code below. It will export usernames and their MFA status to a CSV file.
# Read and process the CSV file $Users = Import-Csv -Path <CSVFilePath> $OutputCSVName = ".\MFAreport_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" foreach ($User in $Users) { $UserPrincipalName = $User.UPN $UserMFAStatus = Get-MgBetaUserAuthenticationMethod -UserId $UserPrincipalName # Determine MFA status $MfaStatus = if ($UserMFAStatus.Count -gt 1) { "Enabled" } else { "Disabled" } $Result = [PSCustomObject]@{ UPN = $UserPrincipalName "MFA Status" = $MfaStatus } # Export the result to a CSV file $Result | Export-Csv -Path $OutputCSVName -NoTypeInformation -Append }
MSOnline:
If you want to use thePoweShell cmdlet Get-MSolUser to check the MFA status, use the below code.
# Define the path for the input CSV file $Users = Import-Csv -Path <CSVFilePath> # Define the path for the output CSV file with a timestamp $outputCSVName = ".\MFAreport_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" foreach ($user in $users) { $userPrincipalName = $user.UPN $mfaStatus = (Get-MsolUser -UserPrincipalName $userPrincipalName).StrongAuthenticationRequirements.state # Determine MFA status if ($mfaStatus -eq $null) { $mfaStatus = "Disabled" } $result = [PSCustomObject]@{ UserPrincipalName = $userPrincipalName "MFA Status" = $mfaStatus } # Export the result to the output CSV file with -Append $result | Export-Csv -Path $outputCSVName -NoTypeInformation -Append }
Using this use case, administrators can gain a comprehensive view of the MFA status for all Microsoft 365 users in their organization. By exporting an MFA status report, they can assess the level of security, compliance, and identify users who have or have not enabled MFA.
MS Graph:
To generate Azure MFA report (Currently, Entra ID MFA report) for all the Microsoft 365 users, run the following PowerShell script.
$OutputCSVName = ".\MFAStatusReport_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" Get-Mguser -All | foreach { $UPN = $_.UserPrincipalName Write-Progress -Activity "`n Retrieving MFA status "`n" Currently Processing: $UPN" $UserMFAStatus = Get-MgBetaUserAuthenticationMethod -UserId $UPN # Determine MFA status $MfaStatus = if ($UserMFAStatus.Count -gt 1) { "Enabled" } else { "Disabled" } $Result = [PSCustomObject]@{ "UPN" = $UPN "MFA Status" = $MfaStatus } # Export the result to a CSV file $Result | Export-Csv -Path $OutputCSVName -NoTypeInformation -Append }
MSOnline:
If you want to export M365 users and their MFA status using the Get-MSolUser cmdlet, you can use the below code snippet.
$OutputCSVName = ".\MFAStatusReport_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" Get-MsolUser -all | select UserPrincipalName,@{Name=”MFA Status”; E={if( $_.StrongAuthenticationRequirements.State -ne $null){ $_.StrongAuthenticationRequirements.State} else {“Disabled”}}} | Export-Csv -Path $OutputCSVName -NoTypeInformation –Append
This report helps administrators quickly identify and confirm the MFA enabled users.
MS Graph:
To identify users with MFA, execute the PowerShell script below.
$OutputCSVName = ".\UsersWithMFA_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" Get-Mguser -All | foreach { $UPN = $_.UserPrincipalName Write-Progress -Activity "`n Retrieving MFA status "`n" Currently Processing: $UPN" $UserMFAStatus = Get-MgBetaUserAuthenticationMethod -UserId $UPN # Determine MFA status if ($UserMFAStatus.Count -gt 1) { $MfaStatus ="Enabled" $Result = [PSCustomObject]@{ "UPN" = $UPN "MFA Status" = $MfaStatus } # Export the result to a CSV file $Result | Export-Csv -Path $OutputCSVName -NoTypeInformation -Append } }
Note: You can also use this method to identify users’ MFA status enabled through Conditional Access policies.
MSOnline:
To generate per-user MFA enabled users, run the following script.
$OutputCSVName = ".\MFAEnabledUsersReport_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" Get-MsolUser -All | foreach { $UPN = $_.UserPrincipalName Write-Progress -Activity "`n Retrieving MFA status "`n" Currently Processing: $UPN" $UserMFAStatus = $_.StrongAuthenticationRequirements.State if ($UserMFAStatus -ne $null) { $Result = [PSCustomObject]@{ "UPN" = $UPN "MFA Status" = $UserMFAStatus } # Export the result to a CSV file $Result | Export-Csv -Path $OutputCSVName -NoTypeInformation -Append } }
By referring to these reports, admins can effectively disable MFA for a specific user account using the CA policy based on their requirements.
This report lists users who have not configured MFA for their accounts. It helps to identify users who may need to enable MFA to strengthen their account protection.
MS Graph:
To find users without MFA, run the following script.
$OutputCSVName = ".\MFADisabledUsers_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" Get-Mguser -All | foreach { $UPN = $_.UserPrincipalName Write-Progress -Activity "`n Retrieving MFA status "`n" Currently Processing: $UPN" $UserMFAStatus = Get-MgBetaUserAuthenticationMethod -UserId $UPN # Determine MFA status if ($UserMFAStatus.Count -eq 1) { $Result = [PSCustomObject]@{ "Users without MFA" = $UPN } $Result | Export-Csv -Path $OutputCSVName -NoTypeInformation -Append } }
MSOnline:
If your organization has adopted per-user MFA, you can find user accounts not protected by MFA using the script below.
$OutputCSVName = "D:\MFADisabledUsersReport_$((Get-Date -format yyyy-MMM-dd-ddd` hh-mm` tt).ToString()).csv" Get-MsolUser -All | foreach { $UPN = $_.UserPrincipalName Write-Progress -Activity "`n Retrieving MFA status "`n" Currently Processing: $UPN" $UserMFAStatus = $_.StrongAuthenticationRequirements.State if ($UserMFAStatus -ne $null) { $Result = [PSCustomObject]@{ "Users Without MFA" = $UPN } # Export the result to a CSV file $Result | Export-Csv -Path $OutputCSVName -NoTypeInformation -Append } }
Administrators often find managing MFA through PowerShell cmdlets to be a complex task. To alleviate this challenge, the AdminDroid Microsoft 365 reporting tool provides a diverse array of reports for streamlined monitoring of MFA status and sign-ins. This includes:
-
- MFA Status Reports:
- Users with MFA
- Users without MFA
- MFA enabled & enforced users
- MFA disabled users
- MFA activated & non-activated users
- MFA device details
- Admins without MFA
- MFA Audit Reports:
- Audit when MFA is enabled
- Audit when MFA is disabled
- MFA Analytics Reports:
- Users categorized by MFA status
- Users categorized by default authentication methods
- Users categorized by configured authentication methods
- MFA Sign-in Analytics Reports:
- Failed MFA challenge sign-ins
- All successful MFA sign-ins
- MFA sign-ins via text message
- MFA sign-ins via phone call
- MFA sign-ins via app notification
- MFA sign-in via app verification
- MFA Source Reports:
- MFA sign-ins through Conditional Access policies
- MFA sign-ins through user-level settings
- MFA Status Reports:
Furthermore, the MFA status dashboard provides a clear view of your organization’s security exposure. AdminDroid doesn’t stop there; it offers over 1800 pre-built reports and 30+ smart dashboards covering various Microsoft services, allowing you to gain in-depth insights.
Download AdminDroid’s Microsoft 365 administration tool now to efficiently manage your M365 environment and enhance your security posture.
I hope this blog will help administrators efficiently monitor MFA status for their users and configure MFA to bolster overall security..